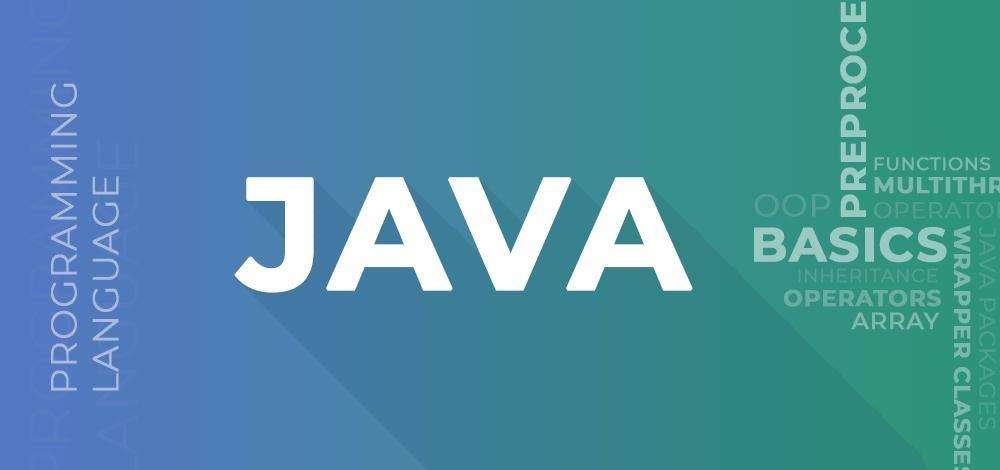
Java stands as a colossus in the world of programming, its robust structure supporting the digital framework of countless enterprises. This tutorial is your compass in navigating the Java landscape, a roadmap to mastering a language that has shaped the technological Area.
This Java guide is crafted for both newcomers and seasoned experts. If you’re aiming to grasp Java’s fundamentals or dive into its more complex features, this complimentary Java guide is an ideal choice for your learning journey.
Java, the cornerstone of modern software development, beckons beginners and seasoned coders alike to dive into its depths. It’s a language that offers a blend of performance, reliability, and versatility, making it a preferred choice for everything from mobile applications to large-scale enterprise systems.
What is Java?
Sun Microsystems introduced Java in 1995, and it quickly rose to fame as an object-oriented language. This versatile language is key for Android apps, web services, AI, cloud-based solutions, and beyond.
This guide will walk you through Java’s syntax essentials to sophisticated concepts such as object-oriented design and error management. By the conclusion of this guide, you’ll be well-equipped with Java knowledge, poised to craft your own applications. Let’s embark on this detailed journey into Java programming!
Free Java Online Compiler
// A Java program to print “Hello World”
Public class GFG {
Public static void main(String args[])
{
System.out.println(“Hello World”);
}
}
Output:
Hello World
The overview of Java Roadmap & Tutorial
¡》Why Java?
Java’s philosophy of “Write Once, Run Anywhere” (WORA) ensures that Java applications are portable across platforms, a feature that has cemented its popularity. Its object-oriented nature promotes clean, modular code, and its vast ecosystem of libraries and frameworks accelerates development and innovation.
¡¡》Setting Up Your Environment
Before embarking on your Java journey, setting up a proper development environment is crucial. This involves installing the Java Development Kit (JDK) and an Integrated Development Environment (IDE) like Eclipse or IntelliJ IDEA, which provide comprehensive tools for code writing, testing, and debugging.
¡¡¡》Understanding the Basics
Begin with the basics: data types, variables, operators, and control structures. Grasp the syntax that is the foundation of Java programming. Experiment with simple programs that teach you to input and output data, manipulate it, and understand the flow of a Java program.
¡V》Object-Oriented Programming
Dive into the core of Java—object-oriented programming (OOP). Learn about classes, objects, inheritance, polymorphism, and encapsulation. These concepts are not just the backbone of Java, but they also form the basis of modern software design.
V》Advanced Features
Once you’re comfortable with the basics, explore advanced features like lambda expressions, streams, and the Java Collections Framework. These powerful tools will enable you to write more efficient and concise code.
V¡》Building Applications
Move beyond theory and start building real-world applications. Begin with console applications, then advance to graphical user interfaces (GUIs) using Swing or JavaFX. Tackle web development with servlets and JSPs, and understand how Java serves as the backend for many web services.
V¡¡》The Road Ahead
The journey doesn’t end here. The Java ecosystem is ever-evolving, with new frameworks like Spring and Hibernate revolutionizing how we approach enterprise application development. Keep learning, keep coding, and stay updated with the latest trends in the Java community.
Java Programming Related Resources
- Recent Articles on Java
- Java Programs – Basics to Advanced
- Java Interview Questions
Get Started with Free Java Tutorial
In this section, you’ll discover a wealth of complimentary materials to guide you from beginner to expert in the Java programming language.
Features of Java
Certainly! Here’s the information presented in bullet points:
- Java is renowned and extensively utilized.
- It has consistently been a top choice among programming languages.
- Java is object-oriented, but not purely, due to support for primitives like `int`, `char`.
- Java code compiles to bytecode, which runs on the Java Virtual Machine (JVM), architecture-independent.
- Java’s syntax echoes C/C++, but without low-level functionalities such as pointers.
- Java requires coding in classes and objects.
- It’s applied in mobile (Android), desktop, web, client-server, and enterprise applications.
- Java is more maintainable than C++, avoiding practices leading to inefficient programming (e.g., no passing of large objects, only references).
- Java eliminates bad memory access risks by not using pointers.
- Java sits between C++ and Python: faster than Python, slower than C++, with static type checking unlike Python’s dynamic checking.
Applications of Java
In this section, we highlight a variety of applications crafted with Java:
Mobile Applications: Java is a primary language for Android app development, offering a rich API and robust environment.
Desktop GUI Applications: Java provides GUI development capabilities through Swing and JavaFX, enabling the creation of sophisticated desktop applications.
Artificial Intelligence: Java’s scalability and robust library ecosystem make it suitable for AI projects, including machine learning and neural networks.
Scientific Applications: Java’s stability and security features are ideal for scientific computing and complex calculations.
Cloud Applications: With its portability and robustness, Java is often used for building scalable cloud applications.
Embedded Systems: Java’s platform independence allows it to run on various embedded systems, from consumer devices to complex control systems.
Gaming Applications: Java’s versatility and performance make it a choice for developing cross-platform games.
These applications showcase Java’s flexibility and its ability to adapt to different technological needs.
Prerequisites to Learn Java
In this article, we’ve curated our finest resources to simplify your learning of Java’s core concepts. However, it’s advisable for readers to have a basic understanding of programming principles. This foundational knowledge will help you quickly grasp Java’s variables, commands, syntax, and more.
Complete Java Tutorial & Roadmap
In this section, you will find all the free resources that you need to become zero to mastery in Java programming language. Let’s get Started.
1. Overview of Java
- Introduction to Java
- History of Java
- Java vs C++ Python
- How to Download and Install Java?
- Setting Up the Environment in Java
- How to Download and Install Eclipse on Windows?
- Java Development Kit (JDK) in Java
- JVM and its architecture
- Differences between JDK, JRE, and JVM
- Just In Time Compiler
- Difference Between JIT and JVM
- Difference Between Byte Code and Machine Code
- How is the Java platform independent?
2. Basics of Java
- Java Basic Syntax
- First Java Program (Hello World)
- Datatypes in Java
- Difference between Primitive and Non-Primitive Datatypes
- Java Identifiers
- Operators in Java
- Java Variables
- Scope of Variables
- Wrapper Classes in Java
3. Input/Output in Java
- How to take Input from users in Java
- Scanner class in Java
- BufferedReader class in Java
- Scanner vs BufferedReader in Java
- Ways to Read Input from Console in Java
- Print Output in Java
- Difference between print() and println() in Java
- Formatted Outputs in Java
- Fast Input-Output for Competitive Programming in Java
4. Flow Control in Java
- Decision making in Java
- If Statement in Java
- If-Else Statement in java
- If-Else-If ladder in Java
- Loops in Java
- For loop
- While Loop
- Do while loop
- For each loop
- Continue Statement in java
- Break Statement In Java
- Usage of Break in Java
- Return Statement in Java
4. Operators in Java
- Arithmetic Operator
- Unary Operator
- Assignment Operator
- Relational Operator
- Logical Operator
- Ternary Operator
- Bitwise Operator
5. Strings in Java
- Introduction of Strings in Java
- String class in Java Set-1 | Set-2
- Why strings are immutable in Java?
- StringBuffer class in Java
- StringBuilder class in Java
- Strings vs StringBuffer vs StringBuilder in Java
- StringTokenizer class in Java Set-1 | Set-2
- StringJoiner in Java
- Java String Programs
6. Arrays in Java
- Introduction to Arrays in Java
- Arrays class in Java
- Multi-Dimensional Array in Java
- How to declare and initialize 2D arrays in Java
- Jagged array in Java
- Final Arrays in Java
- Reflect Arrays in Java
- Difference between util.Arrays and reflect.Arrays
- Java Array Programs
7. OOPS in Java
- OOPS Concept in Java
- Why Java is not a purely Object-Oriented Language?
- Classes and Objects
- Naming Convention in Java
- Methods in Java
- Access Modifiers in Java
- Constructors in Java
- Four pillars of OOPS in Java
8. Inheritance in Java
- Abstraction in Java
- Encapsulation in Java
- Polymorphism in Java
- Interfaces in Java
- This reference in Java
- Inheritance in Java
- Introduction to Inheritance in Java
- Inheritance and Constructors
- Multiple Inheritance in Java
- Interfaces and Inheritance
- Association, Composition and Aggregation
- Difference between Inheritance in C++ and Java
9. Abstraction in Java
- Introduction to Abstraction in Java
- Abstract Keyword in Java
- Abstract classes in Java
- Abstract class vs Interface in Java
- Control Abstraction in Java
- Difference between Data Hiding and Abstraction
10. Encapsulation in Java
- Introduction to Encapsulation in Java
- Difference between Encapsulation and Abstraction
11. Polymorphism in Java
- Introduction to Polymorphism in Java
- Difference between Inheritance and Polymorphism
- Runtime Polymorphism in Java
- Compile-Time vs Runtime Polymorphism
12. Constructors in Java
- Introduction to Constructors in Java
- Copy Constructor in Java
- Constructor Overloading
- Constructor Chaining
- Private Constructors and Singleton Class
13. Methods in Java
- Introduction to methods in Java
- Different method calls in Java
- Difference between Static methods and Instance methods in Java
- Abstract methods in Java
- Method Overriding in Java
- Method Overloading in Java
- Method Overloading Vs Method Overriding
14. Interfaces in Java
- Java Interfaces
- Interfaces and Inheritance in Java
- Difference between Interface and Class in Java
- Functional Interface
- Nested Interface
- Marker Interface
- Comparator Interface
15. Wrapper Classes in Java
- Need of Wrapper classes in Java
- How to create instances of Wrapper classes
- Character class in Java
- Byte class in Java
- Short class in Java
- Integer class in Java
- Long class in Java
- Float class in Java
- Double class in Java
- Boolean class in Java
- Autoboxing and Unboxing
- Type Conversion in Java
16. Keywords in Java
- List of all Java Keywords
- Important Keywords in Java
- Super Keyword
- Final Keyword
- Abstract keyword
- Static Keyword
- This Keyword
- Enum Keyword in Java
- Transient keyword in java
- Volatile keyword in java
- Final, Finally, and Finalize in Java
17. Access Modifiers in Java
- Introduction to Access Modifiers in Java
- Public vs Protected vs Package vs Private Access Modifier in Java
- Access Modifiers Vs Non-Access Modifiers in Java
18. Memory Allocation in Java
- Java Memory Management
- How are Java objects stored in memory
- Stack vs Heap memory allocation
- Types of memory areas allocated by JVM
- Garbage Collection in Java
- Heap and Stack memory allocation
- Types of JVM Garbage Collectors in Java
- Memory leaks in Java
- Java Virtual Machine(JVM) Stack Area
19. Classes of Java
- Classes and Objects
- Understanding classes and objects in Java
- Class vs interface
- Singleton class in java
- Object class in java
- Inner class in java
- Abstract classes in java
- Throwable class in java
20. Packages in Java
- Java Packages
- How to create a package in Java
- Java.util package
- Java.lang package
- Java.io package
21. Collection Framework in Java
- Java Collection Framework
- Collections class in Java
- Collection Interface in Java
- How to learn Java collections
- List Interface in Java
- Queue Interface in Java
- Map Interface in Java
- Set in Java
- Iterator in Java
- Comparator in Java
- Difference between Comparator and Comparable in Java
22. List in Java
- ArrayList in Java
- Vector class in Java
- Stack class in Java
- LinkedList in Java
- AbstractList
- AbstractSequentialList
- CopyOnWriteArrayList
- Custom ArrayList in Java
23. Queue in Java
- AbstractQueue
- ArrayBlockingQueue
- ConcurrentLinkedQueue
- LinkedBlockingQueue
- LinkedTransferQueue
- PriorityBlockingQueue
- Deque in Java
- ArrayDeque
- Concurrent LinkedDeque
- LinkedBlocking Deque
- Priority Queue in Java
24. Map in Java
- EnumMap
- HashMap
- Working of HashMap
- Traverse through a HashMap in Java
- WeakHashMap
- LinkedHashMap
- IdentityHashMap
- ConcurrentHashMap
- Dictionary
- HashTable
- SortedMap
- TreeMap
- Stack
- Vector
25. Set in Java
- AbstractSet
- EnumSet
- HashSet
- TreeSet
- SortedSet
- LinkedHashSet
- NavigableSet
- ConcurrentSkipListSet
- CopyOnWriteArraySet
24. Exception Handling in Java
- Exceptions in java
- Types of Exceptions
- Difference between Checked and Unchecked Exceptions
- Try, Catch, Finally, throw, and throws
- Flow control in Try catch block
- Throw vs Throws
- Final vs Finally vs Finalize
- User-defined custom exception
- Chained Exceptions
- Null pointer Exceptions
- Exception handling with method Overriding
25. Multithreading in Java
- Introduction to Multithreading in Java
- Lifecycle and Stages of a Thread
- Thread Priority in Java
- Main Thread in Java
- Thread class
- Runnable interface
- How to name a thread
- Start() method in thread
- Difference between run() and start() Method
- Sleep() method
- Daemon thread
- Thread Pool in Java
- Thread Group in Java
- Thread Safety in Java
- ShutdownHook
- Multithreading Tutorial
26. Synchronization in Java
- Java Synchronization
- Importance of Thread synchronization in Java
- Method and Block Synchronization in Java
- Local frameworks vs thread synchronization
- Difference between Atomic, Volatile, and Synchronized in Java
- Deadlock in Multithreading
- Deadlock Prevention and Avoidance
- Difference between Lock and Monitor in Concurrency
- Reentrant Lock
27. File Handling in Java
- File Class in java
- How to create files in java
- How to read files in java
- How to write on files in java
- How to delete a file in java
- File Permissions
- FileReader
- File Writer
- FileDescriptor class
- RandomAccessFile class
28. Java Regex
- Introduction to Java Regex
- How to write Regex expressions
- Matcher class
- Pattern class
- Quantifiers
- Character class
29. Java IO
- Introduction to Java IO
- Reader Class
- Writer Class
- FileInput stream
- File Output stream
- BufferedReader Input Stream
- BufferedReader Output stream
- BufferedReader vs Scanner
- Fast I/O in Java
30. Java Networking
- Introduction to Java Networking
- TCP architecture
- UDP architecture
- IPV4 vs IPV6
- Connection-oriented vs connectionless protocols
- Socket programming in Java
- Server Socket class
- URL class and methods
31. Java SE 8 Features
- Lambda Expressions
- Streams API
- New Date/Time API
- Default Methods
- Functional Interfaces
- Method references
- Optional class
- Stream Filter
- Type Annotations
- String Joiner
32. Java Date & Time
- Date Class in Java
- Methods of the Date class
- Java Current Date and time
- Compare dates in Java
33. Java JDBC
- Introduction to Java JDBC
- JDBC Driver
- JDBC Connection
- Types of Statements in JDBC
- JDBC Tutorial
34. Java Miscellaneous
- Introduction to Reflection API
- Java IO Tutorial
- JavaFX Tutorial
- Java RMI
- How to Run Java RMI application?
- Java 17 New Features
- Interview Questions on Java
- Core Java Interview Questions
- Java Multiple Choice Questions
35. Interview Questions on Java
- Core Java Interview Questions
- Java Multiple Choice Questions
Java Programing Language Quiz
- Quizzes on Java Language
If you Prefer a course,
And why go anywhere else when our Java Programming complete master class From Beginner to Advanced Course helps you do this in a single program! Apply now to our Java course and our counsellors will connect with you for further guidance & support.
Conclusion
Java’s roadmap is vast and filled with opportunities for growth and innovation. Whether you’re starting your programming journey or looking to enhance your skill set, Java offers a path that can lead to a fulfilling career in technology. Embrace the journey, and let Java unlock doors to a world of possibilities.
RELATED ARTICLES
- HTML Complete Course Guide From Beginner to Advanced Levels
- HTML Tutorial & Roadmap
- CSS Tutorial & Roadmap
- CSS Complete Guide – From Novice to Pro CSS Concepts
- JavaScript Complete Guide From Beginner to Advanced Levels
- A Comprehensive JavaScript Tutorial & Roadmap
- Complete React Tutorial & Roadmap
- Complete NodeJS Tutorial & Roadmap
- C Programming Language Tutorial & Roadmap
- The Ultimate C++ Programming Language Tutorial & Roadmap
- Complete Ruby Programming Language Guide
- Complete Perl Programming Language Guide
- R Tutorial & Roadmap| Master R Programming with Ease
- PHP Tutorial & Roadmap: A Comprehensive Guide for Developers
- Golang (Go) Programming Tutorial & Roadmap: Go Beyond the Basics
- A Comprehensive SQL Tutorial & Roadmap